Qt5 Signal Slot Tutorial
- Qt5 Signal Slot Example
- Qt Signal Slot Tutorial
- Qt5 Signal Slot Tutorial For Beginners
- Qt5 Signal Slot Tutorial Python
- Qt5 Signal Slot Tutorial Arduino
- Qt5 Signal Slot Tutorial Android Studio
The signal on its own does not perform any action. Instead, it is ‘connected’ to a ‘slot’. The slot can be any callable Python function. In PyQt, connection between a signal and a slot can be achieved in different ways. Following are most commonly used techniques − QtCore.QObject.connect(widget, QtCore.SIGNAL(‘signalname’), slot. Nd the index of the signal and of the slot Keep in an internal map which signal is connected to what slots When emitting a signal, QMetaObject::activate is called. It calls qt metacall (generated by moc) with the slot index which call the actual slot. This signal does nothing, by itself; it must be connected to a slot, which is an object that acts as a recipient for a signal and, given one, acts on it. Connecting Built-In PySide/PyQt Signals. Qt widgets have a number of signals built in. For example, when a QPushButton is clicked, it emits its clicked signal.
Graphical applications (GUI) are event-driven, unlike console or terminal applications. A users action like clicks a button or selecting an item in a list is called an event.
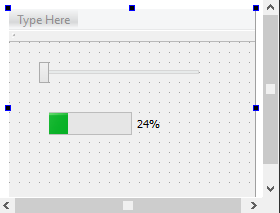
If an event takes place, each PyQt5 widget can emit a signal. A signal does not execute any action, that is done by a slot.
Related course:
Create GUI Apps with PyQt5
Signals and slot introduction
Consider this example:
The button click (signal) is connected to the action (slot). In this example, the method slot_method will be called if the signal emits.
This principle of connecting slots methods or function to a widget, applies to all widgets,
or we can explicitly define the signal:
PyQt supports many type of signals, not just clicks.
Example
We can create a method (slot) that is connected to a widget. A slot is any callable function or method.
On running the application, we can click the button to execute the action (slot).
If you are new to programming Python PyQt, I highly recommend this book.
A simple Qt 5 Hello World tutorial that demonstrates how to use Qt Creator to make a GUI application window. Create a window with two text labels and a button in this easy tutorial for beginners. Program the application in C++.
The image below shows the finished Qt GUI window application. When the button is clicked, the text “Hello, world!” is displayed in the first text label. Each time the button is clicked, the count in the second label is incremented.
Part 5 of the Qt Creator C++ Tutorial
Qt 5 Hello World Tutorial Steps
The following steps show how to create the Qt5 Hello World application using Qt Creator. Before continuing, be sure to install Qt Creator and other required software packages.
1. Start a New Qt Creator Project
Open the Qt Creator application and click the New Project button, or File → New File or Project … from the top menu.
In the New Project dialog box choose Qt Widgets Application and then click the Choose… button.
In the next dialog box enter the name of the project and choose the location to create the project in. Click the Next > button when done.
The Qt Kit can now be selected. If this is the first time using Qt Creator, you may be prompted to set up a kit. If a kit is already selected, it can be modified by hovering the mouse cursor over the kit name and then clicking the Manage… button that appears after hovering the mouse cursor, e.g. over Desktop in the below image.
Click the Next > button and leave the class information at the default values.
Click the Next > button and then the Finish button.
At this stage the Run button can be clicked to build and run the new Qt Creator application (or use Ctrl + R on the keyboard to run). When the application runs, a window will appear that can be resized and closed.
2. Edit the Application Window Graphically
To start editing the new application window in Qt Creator, expand the Forms folder at the top left of Qt Creator. Double-click the mainwindow.ui file that appears. Qt Creator will now change to Design mode allowing the hello application window to be edited.
2.1 Delete Unneeded Widgets from the Main Window
The default Qt Creator application window has a menu bar, tool bar and status bar. These widgets are not needed in this simple Hello World application, so they can be deleted. Each widget in the main window form has corresponding text at the top right of Qt Creator. This makes it easy to delete these widgets as they are difficult to see on the window form when they are empty.
Right-click the text for each widget at the right of Qt Creator and then click Remove on the menu that pops up to delete the widget from the window form.
2.2 Add Text Labels
Drag and drop two Label widgets from the pane at the left of the window form, under Display Widgets. Drop the labels onto the form.
2.3 Size the Text Labels
Click one of the text labels on the form, then hold down the Ctrl key and click the second label to select both of them. Now click the Lay Out Vertically button on the toolbar above the form or press Ctrl + L on the keyboard. This will allow both text labels to be resized at the same time while keeping them aligned. Resize the text labels making them longer by dragging on one of the square handles around the labels. The labels can also be moved to the desired position on the window form.
2.4 Middle Align the Label Text
Text in both labels can be aligned to the middle of the label box by first selecting both labels. Be sure to click one label, hold down the Ctrl key and then click the other label. This is different than selecting the vertical layout box added for aligning and sizing the text boxes in the previous step.
In the bottom right pane of Qt Creator, scroll down to find alignment under QLabel. If the alignment property is not expanded, expand it by clicking the arrow at the left of it. Now click in the value field to the right of Horizontal and select AlignHCenter. Both text labels will now be center aligned.
2.5 Change the Label Name and Text
Qt5 Signal Slot Example
Click the top label on the window form and then change the label name next to objectName under QObject in the right bottom pane. Change the label name to lblHello. Change the label text to … next to text under QLabel.
Do the same for the second label, but change the label name to lblCount.
2.6 Add and Edit a Button Widget
Add a Push Button to the window form by dragging and dropping it from the left widget pane under Buttons.
Make sure that the button is selected on the form. Change the name of the button to btnHello and the text of the button to Hello in the same way as the labels were edited.
3. Connecting the Button to Code
We now want to connect the button to the code that will run when it is clicked. Right-click the button on the form and select Go to slot… on the menu that pops up. Choose clicked() in the dialog box that pops up and then click the OK button.
Qt Creator will now switch to the member function that will be called when the button is clicked.
4. Application Operational Code
The application code can now be written. The code writes “Hello, world!” to the first text label in the window and increments a count in the second text label every time that the button is clicked.
The code listing below shows the code added to the button click handler in the mainwindow.cpp file.
Static integer count holds the count value that is incremented on each button click. The first time that the application runs, both labels will contain the text … which will be replaced by the “Hello, world!” text message and the incrementing count.
Each label is accessed by its name that was set in the GUI editor. Widgets are all accessed as member pointers of the user interface object (ui) and so pointer notation is used, e.g. ui->widget name
5. Change Window Size and Text
To switch back to editing the window form, click mainwindow.ui at the bottom left of Qt Creator under Open Documents. Qt Creator will change back to Design mode.
Qt Signal Slot Tutorial
5.1 Change Window Size
Qt5 Signal Slot Tutorial For Beginners
Click the main window form and use the sizing handles to size the window to the desired size. The window size can also be changed by selecting the window and changing the Width and Height under QWidget and geometry in the bottom right Qt Creator pane.
Qt5 Signal Slot Tutorial Python
5.2 Change Window Title Text
With the window form selected in Qt Creator, find windowTitle under QWidget in the bottom right pane of Qt Creator. In the image below, the window title was changed to “Hello Application”. This text will now appear in the top title bar of the application window when it is run.
6. Build and Run the Application
Save changes to the project. This can be done using File → Save All from the top menu or Ctrl + Shift + S on the keyboard.
Qt5 Signal Slot Tutorial Arduino
The Qt 5 Hello World Tutorial project can be built by clicking the hammer icon on the left toolbar of Qt Creator. The application can be run by clicking the green triangle icon on the left toolbar.
Qt5 Signal Slot Tutorial Android Studio
Qt 5 Hello World Tutorial Development Environment
This tutorial was developed on a Linux Mint 18 Mate 64-bit computer using Qt Creator 3.5.1 and Qt 5.5.1. Compiled with g++ compiler version 5.4.0.