Signal Slot Python Qt
Although PyQt4 allows any Python callable to be used as a slot when connecting signals, it is sometimes necessary to explicitly mark a Python method as being a Qt slot and to provide a C signature for PyQt4 provides the pyqtSlot function decorator to do this. Traditional syntax: SIGNAL and SLOT QtCore.SIGNAL and QtCore.SLOT macros allow Python to interface with Qt signal and slot delivery mechanisms. This is the old way of using signals and slots. The example below uses the well known clicked signal from a QPushButton. It would be possible to have the slots to which the resized and moved signals are connected check the new position or size of the circle and respond accordingly, but it's more convenient and requires less knowledge of circles by the slot functions if the signal that is sent can include that information. PyQt5 signals and slots Graphical applications (GUI) are event-driven, unlike console or terminal applications. A users action like clicks a button or selecting an item in a list is called an event. If an event takes place, each PyQt5 widget can emit a signal.
This section describes the older style for connecting signals and slots. Ituses the same API that a C++ application would use. This has a number ofadvantages.
- It is well understood and documented.
- Any future changes to the C++ API should be easily included.
It also has a number of disadvantages.
- It requires knowledge of the C++ types of signal arguments.
- It is error prone in that if you mis-type the signal name or signature thenno exception is raised, either when the signal is connected or emitted.
- It is verbose.
- It is not Pythonic.
This older style of connecting signals and slots will continue to be supportedthroughout the life of PyQt4.
PyQt4 Signals and Qt Signals¶
Qt signals are statically defined as part of a C++ class. They are referencedusing the QtCore.SIGNAL()
function. This method takes a single stringargument that is the name of the signal and its C++ signature. For example:
The returned value is normally passed to the QtCore.QObject.connect()
method.
PyQt4 allows new signals to be defined dynamically. The act of emitting aPyQt4 signal implicitly defines it. PyQt4 signals are also referenced usingthe QtCore.SIGNAL()
function.
The PyQt_PyObject
Signal Argument Type¶
It is possible to pass any Python object as a signal argument by specifyingPyQt_PyObject
as the type of the argument in the signature. For example:
While this would normally be used for passing objects like lists anddictionaries as signal arguments, it can be used for any Python type. Itsadvantage when passing, for example, an integer is that the normal conversionsfrom a Python object to a C++ integer and back again are not required.
The reference count of the object being passed is maintained automatically.There is no need for the emitter of a signal to keep a reference to the objectafter the call to QtCore.QObject.emit()
, even if a connection is queued.
Short-circuit Signals¶
There is also a special form of a PyQt4 signal known as a short-circuit signal.Short-circut signals implicitly declare each argument as being of typePyQt_PyObject
.
Short-circuit signals do not have a list of arguments or the surroundingparentheses.
Short-circuit signals may only be connected to slots that have been implementedin Python. They cannot be connected to Qt slots or the Python callables thatwrap Qt slots.
PyQt4 Slots and Qt Slots¶
Qt slots are statically defined as part of a C++ class. They are referencedusing the QtCore.SLOT()
function. This method takes a single stringargument that is the name of the slot and its C++ signature. For example:
The returned value is normally passed to the QtCore.QObject.connect()
method.
PyQt4 allows any Python callable to be used as a slot, not just Qt slots. Thisis done by simply referencing the callable. Because Qt slots are implementedas class methods they are also available as Python callables. Therefore it isnot usually necessary to use QtCore.SLOT()
for Qt slots. However, doing sois more efficient as it avoids a conversion to Python and back to C++.
Qt allows a signal to be connected to a slot that requires fewer arguments thanthe signal passes. The extra arguments are quietly discarded. PyQt4 slots canbe used in the same way.
Note that when a slot is a Python callable its reference count is notincreased. This means that a class instance can be deleted without having toexplicitly disconnect any signals connected to its methods. However, if a slotis a lambda function or a partial function then its reference count isautomatically incremented to prevent it from being immediately garbagecollected.
Connecting Signals and Slots¶
Connections between signals and slots (and other signals) are made using theQtCore.QObject.connect()
method. For example:
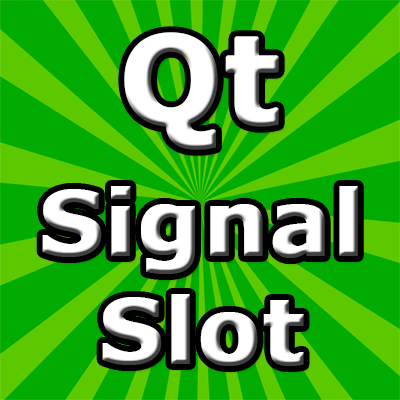
Disconnecting signals works in exactly the same way using theQtCore.QObject.disconnect()
method. However, not all the variations ofthat method are supported by PyQt4. Signals must be disconnected one at atime.
Emitting Signals¶
Any instance of a class that is derived from the QtCore.QObject
class canemit a signal using its emit()
method. This takes a minimum of oneargument which is the signal. Any other arguments are passed to the connectedslots as the signal arguments. For example:
The QtCore.pyqtSignature()
Decorator¶
The QtCore.pyqtSignature()
serves the same purpose as thepyqtSlot()
decorator but has a less Pythonic API.
I'll just give you a short intro to whet your appetite, find all details here yourself.
This is the old way of connecting a signal to a slot. To use the new-style support just replace line 11 with following code
The new-style support introduces an attribute with the same name as the signal, in this case clicked.
Signal Slot Python Qt Download
If you need to define your own signal you'll do something like this (off the top of my head):
Python Qt Signal Slot
And the old way: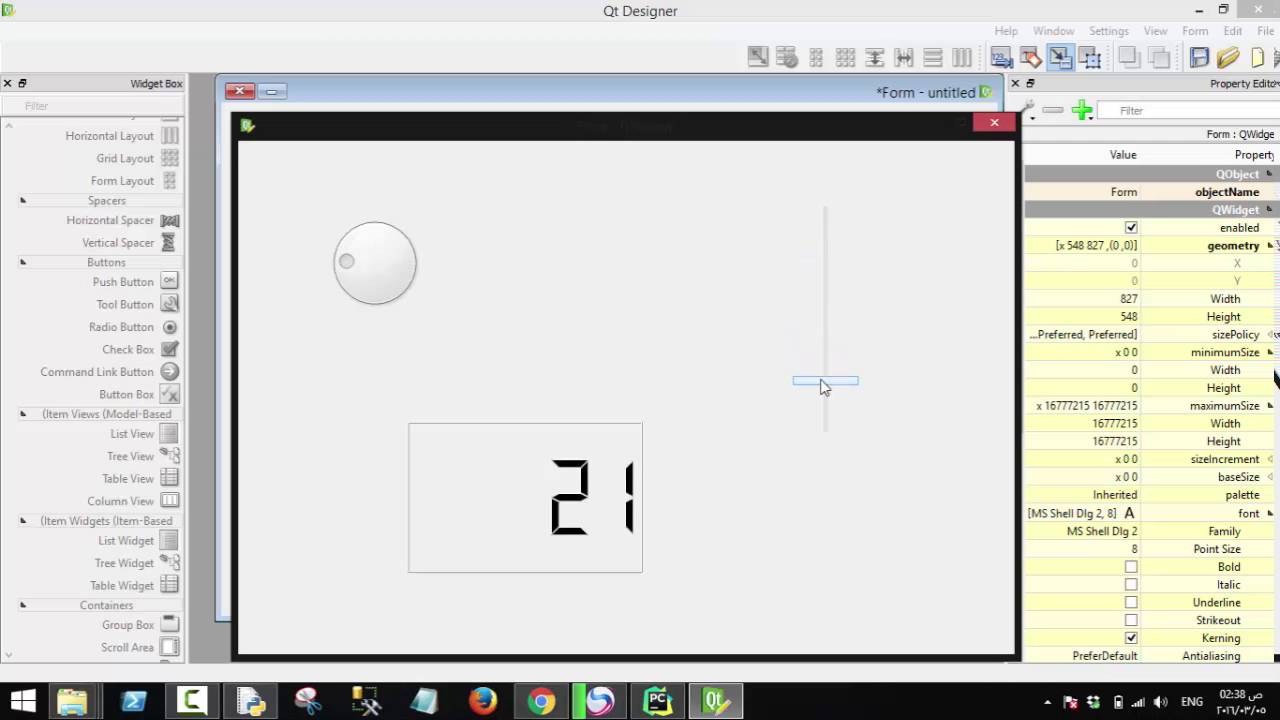